Reactive, responsive, beautiful charts for AngularJS based on Chart.js
We, as front-end developers, will have to go through a lot of javascript frameworks and libraries for bulding your concept in the way you want, making it enormously difficult to choose the right one. But for me it has to be Angular, if there is one framework that has done consistently well over time and you can depend on most of the scenarios.
AngularJS is a Javascript MVC framework created by Google to build properly architectured and maintenable web applications.It defines numerous concepts to properly organize your web application. It enhances HTML by attaching directives to your pages with new attributes or tags and expressions in order to define very powerful templates directly in your HTML.
So Now I’m going to drag your attention on one of the easiest way to visualize your data using Angular, which I found interesting on the first glance itself. Angular Charts…!!
We’ll see how to make beautiful charts using Angular charts plugin with less coding in minimum time. Yes, We can..!!
Chapter 1: Building Your First Chart
For Any project that you are working on , only required dependencies for you to use Angular charts in your work are:
- AngularJS (any minified 1.x will work)
- Chart.js (requires Chart.js 2.0.x).
- Angular Charts Plugin
Why Chart.js ??
Yes Chart.js..
Angular Charts contains a set of native AngularJS directives for Chart.js.
Chart.js is a open-source, light-weight (~11KB) JavaScript library based on the HTML5 canvas control that allows you to draw different types of charts. Since it uses canvas, you have to include a polyfill to support older browsers.
The advantage of using Chart.js is that the charts are responsive, so they will adapt based on the space available. Unlike many libraries you can find on the web, it provides extensive and clear documentation which makes using its basic and advanced features very easy.
Ive explained the process of creating your first chart in the following 4 steps:
Step 1: get your Files ready
Download and Include the dependend files in your index page or the main page of your project using HTML <script> tags. (Make sure you are including these files in the same order as below to avoid dependecy issues)
<script type=”text/javascript” src=”angular.min.js”></script><!– ChartJS library –>
<script src=”path to/Chart.min.js”></script><!– Angular plugin –>
<script src=”path to/angular-chart.min.js”></script>
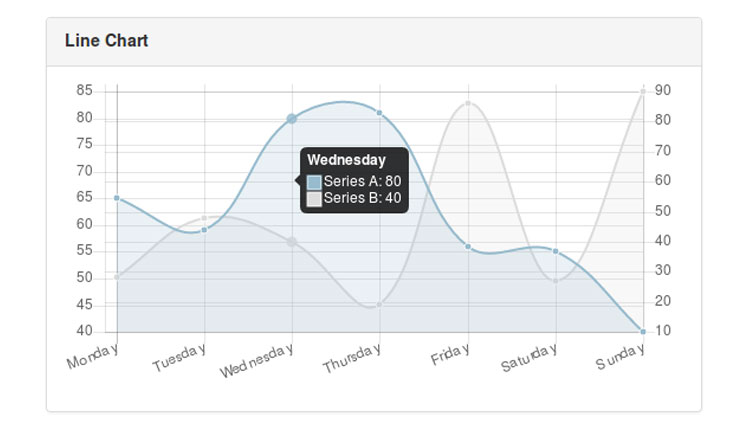
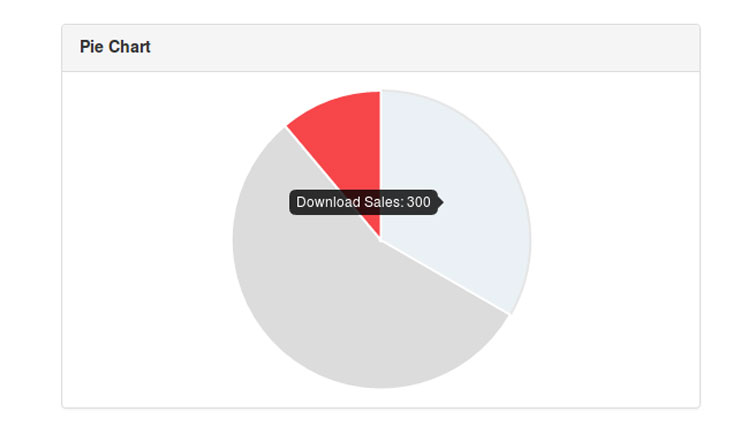
Step-2: Create the AngularJS App
Now you just need to declare a dependency on the chart.js module and inject to your App:
var app = angular.module(‘MychartApp’, [‘angularcharts’]);You can also use the provider : ChartJsProvider in your app.config() to set the default chart colors (Ofcourse you can override these default here: Chart.defaults.global.colors.)
(function (ChartJsProvider) {
ChartJsProvider.setOptions({ colors : [ ‘#803690’, ‘#00ADF9’, ‘#DCDCDC’, ‘#46BFBD’, ‘#FDB45C’, ‘#949FB1’, ‘#4D5360’] });
});
Now you’ve got the canvas and brush in your hands ready…You just need to create your masterpiece..!!
Step 3: Define your controller – “LineCtrl”
Now we will define our first controller for the App- “LineCtrl”. This Controller will be responsible for creating Line Graphs. For this, we augment the controller scope with the configurations for our chart:
app.controller(“LineCtrl”, function ($scope) {
$scope.labels = [“January”, “February”, “March”, “April”, “May”, “June”, “July”];
$scope.series = [‘Series A’, ‘Series B’];
$scope.data = [
[65, 59, 80, 81, 56, 55, 40],
[28, 48, 40, 19, 86, 27, 90]
];
$scope.onClick = function (points, evt) {
console.log(points, evt);
};
$scope.datasetOverride = [{ yAxisID: ‘y-axis-1’ }, { yAxisID: ‘y-axis-2’ }];
$scope.options = {
scales: {
yAxes: [
{
id: ‘y-axis-1’,
type: ‘linear’,
display: true,
position: ‘left’
},
{
id: ‘y-axis-2’,
type: ‘linear’,
display: true,
position: ‘right’
}
]
}
};
});
app.controller(“PieCtrl”, function ($scope) {
$scope.labels = [“Download Sales”, “In-Store Sales”, “Mail-Order Sales”];
$scope.data = [300, 500, 100];
});
Step 4: lets create a chart.
We are almost done now. To render the chart, add Angular Chart directive inside the <canvas> tag where you want to render your chart. This is how we do it:
Angular Charts provides a variety of charts for you like,
– Bar Chart
– Doughnut Chart
– Radar Chart
– Pie Chart
– Polar Area Chart
– Horizontal Bar Chart
– Bubble Chart
– Dynamic Chart etc
the following directives can be used any of these charts:
chart-labels: x axis/series labels
chart-options (default: {}): Chart.js options
chart-series (default: []): series labels
chart-click (optional): onclick event handler
chart-hover (optional): onmousemove event handler
chart-colors (default to global colors): colors for the chart
chart-dataset-override (optional): override datasets individually
chart-type: chart type e.g. Bar, PolarArea, etc for Dynamic Charts
For each chart you’ll have to provide a canvas tag in your html file like :
chart-labels=”labels” chart-series=”series” chart-options=”options”
chart-dataset-override=”datasetOverride” chart-click=”onClick”
</canvas>
In the above code we used:
chart-data : the data arrays that we pass for displaying
chart-labels : labels to be displayed for x Axis.
chart-series : Chart names to be displayed for multiple data sets.(we’ve used series A and series B here)
chart-options : provides the scales options of chart.js
chart-dataset-override : it enables you to override your y axis values for multiple line graphs.
(for more reference go to set- 3)
your chart will look loke this<linechart1.png>
Congrats..!! Now You’ve Created your first chart.
You Can create your pie Chart like this:
(ref “PieCtrl” in step 3)
chart-data=”data” chart-labels=”labels” chart-options=”options”>
</canvas>
<piechart.png>
The same goes for the other charts. click here<http://jtblin.github.io/angular-chart.js> for more options.
Chapter 2: More features on your Charts
Since you know how to build your Angular charts, its time to dig deep into other features like event handlers available in this section.
Fortunately Angular charts provides onclick and onmousehover directives for our charts that deals with the click and hover events.
Remember the function “onclick” in our controller(in step 3),
console.log(points, evt);
};
the points and evnt parameters gives the complete information about the referred point.
The following event handlers are available with Angular Charts:
chart-click : onclick event handler
chart-hover : onmousemove event handler
Now you’ve easily mastered on Angular Charts..!!
Did you find this Section useful? What are the exciting ideas you have got about Angular Charts?
Let us know in the comments.